Configuring Chart.js for SvelteKit: A Comprehensive Tutorial
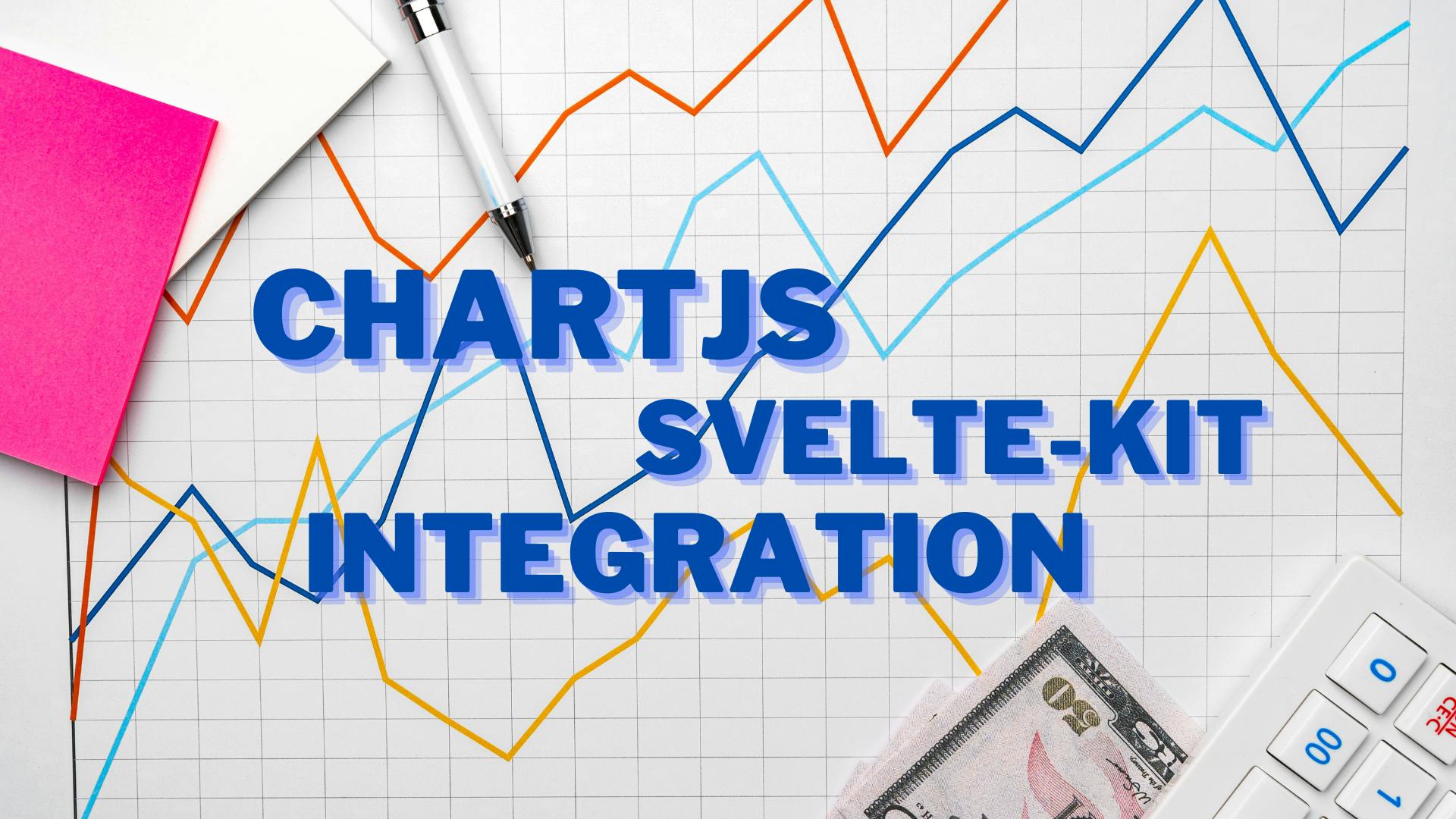
Chart.js is one of the best choices for visualizing data. In this blog post, I'll show you the optimized way to install and configure Chart.js in a SvelteKit project.
To integrate Chart.js, we need to complete a couple of steps:
npm i -D chart.js
chartRender
. It is an arrow function that accepts two parameters. When we use this action, the 'node' parameter will be automatically assigned to the HTML component. //importing chartjs from node_modules
import { Chart } from 'chart.js/auto';
//here we are exporting const variable, which is an arrow function,
//and the arrow function takes one argument called node
export const chartRender = (node, options) => {
console.log('Action')
console.log(node)
console.log(options)
new Chart (node, options)
}
+page.svelte
page.<script>
//importing chartRender from Action file that exported a while ago
import {chartRender} from '../Actions/chartRender'
</script>
<main>
//this is just a simple canvas with action
<canvas use:chartRender>
</canvas>
</main>
Chart.js
. Make sure to export that data so that we can use it in another file.In my case I created a file in lib > data > chartData.js
export const BarData = {
type: 'bar',
data: {
labels: ['January','February', 'March', 'April', 'May'],
datasets: [{
label: 'Bar Dataset',
data: [55,20,30,10,85],
backgroundColor:[
'rgba(245, 40, 145, 0.8)'
]
},],
},
options: {
maintainAspectRatio: false,
},
}
To achieve the desired tasks in the '+page.svelte' file, follow these steps: A. Import the data B. Set the data to the canvas options<script>
import { chartRender } from './../../../lib/Actions/chartRender.js';
import {BarData} from '../../../lib/Data/exp/chartData.js';
</script>
<main class="text-center">
<div class="w-2/5 h-44 border m-auto">
<canvas use:chartRender={BarData}></canvas>
</div>
</main>
Now, we can run npm run dev
and check the result in the browser. At this point, Chart.js should be visible in the browser. At this point our integration is complete. If you need to update the data of Chart.js, you can do so by following the next step: update your 'chartRender.js' file.
Since chartRender.js
serves as the main chart template, we should implement the update and destroy functions inside it. Keep in mind that the update function should be called when we update the Chart.js data, while the destroy function should be called when we hide the Chart.js.
import Chart from '../../../node_modules/chart.js/auto/auto'
export const chartRender = (node, options) => {
console.log("Chart Render is called: ")
const _chart = new Chart (node, options)
return {
update(updatedoptions) {
_chart.data = updatedoptions.data
_chart.update()
},
destroy(){
console.log("Destroy function is called:")
_chart.destroy()
}
}
}