Constructor in JavaScript
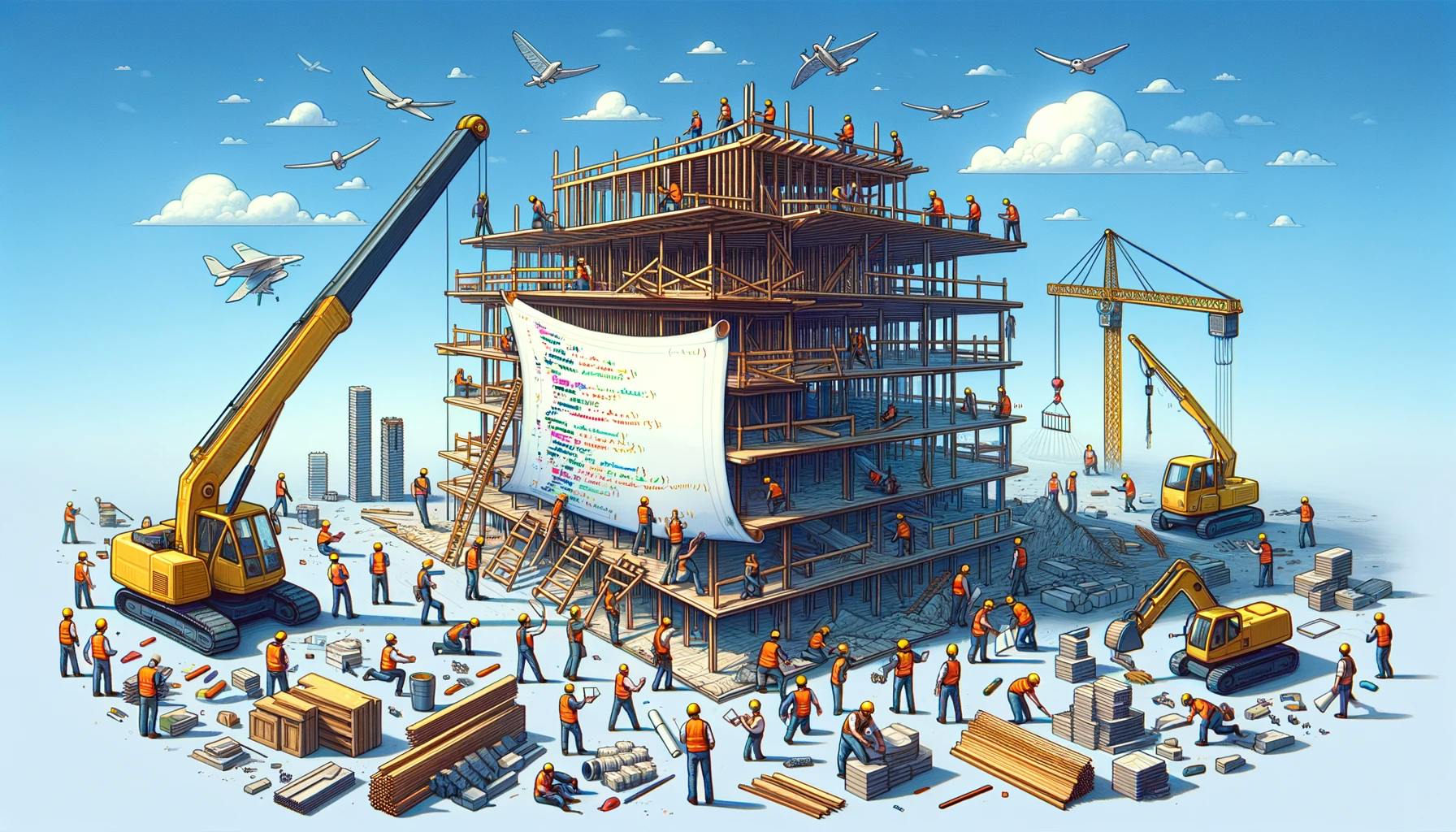
Understanding constructors in JavaScript can be a bit tricky at first, but they're a fundamental part of working with objects and classes in the language.
A constructor in JavaScript is a special function that creates and initializes an object instance of a class. In the context of classical object-oriented programming languages, a constructor is a method that is automatically called when an object is created.
Before ECMAScript 2015 (ES6), JavaScript used constructor functions to simulate the behavior of classes (since JavaScript is prototypal and didn't have a formal class system before ES6). A constructor function is just a regular function, but it follows the convention of starting with a capital letter and is intended to be used with the new keyword.
function Person(name, age) {
this.name = name;
this.age = age;
}
var person1 = new Person("Sabbir", 30);
In this example, Person is a constructor function. When we call it using new Person("Sabbir", 30)
, JavaScript does the following:
Person.prototype
this
set to the new object, and any arguments passed along. ES6 introduced class syntax, which provides a more familiar and clearer syntax for defining constructors and dealing with inheritance. Underneath, it still uses prototypal inheritance, but the syntax is cleaner and more in line with traditional object-oriented languages.
class Person {
constructor(name, age) {
this.name = name;
this.age = age;
}
}
const person1 = new Person("Sabbir", 30);
Here, the constructor method within the Person
class is the constructor. It gets called automatically when a new instance is created using new Person()
this
Keyword: Inside a constructor, the this
keyword refers to the newly created object. Understanding constructors is crucial for effectively using and creating complex objects and classes in JavaScript, allowing for more structured and maintainable code.