Creating Page Route Animation or Transition in Svelte
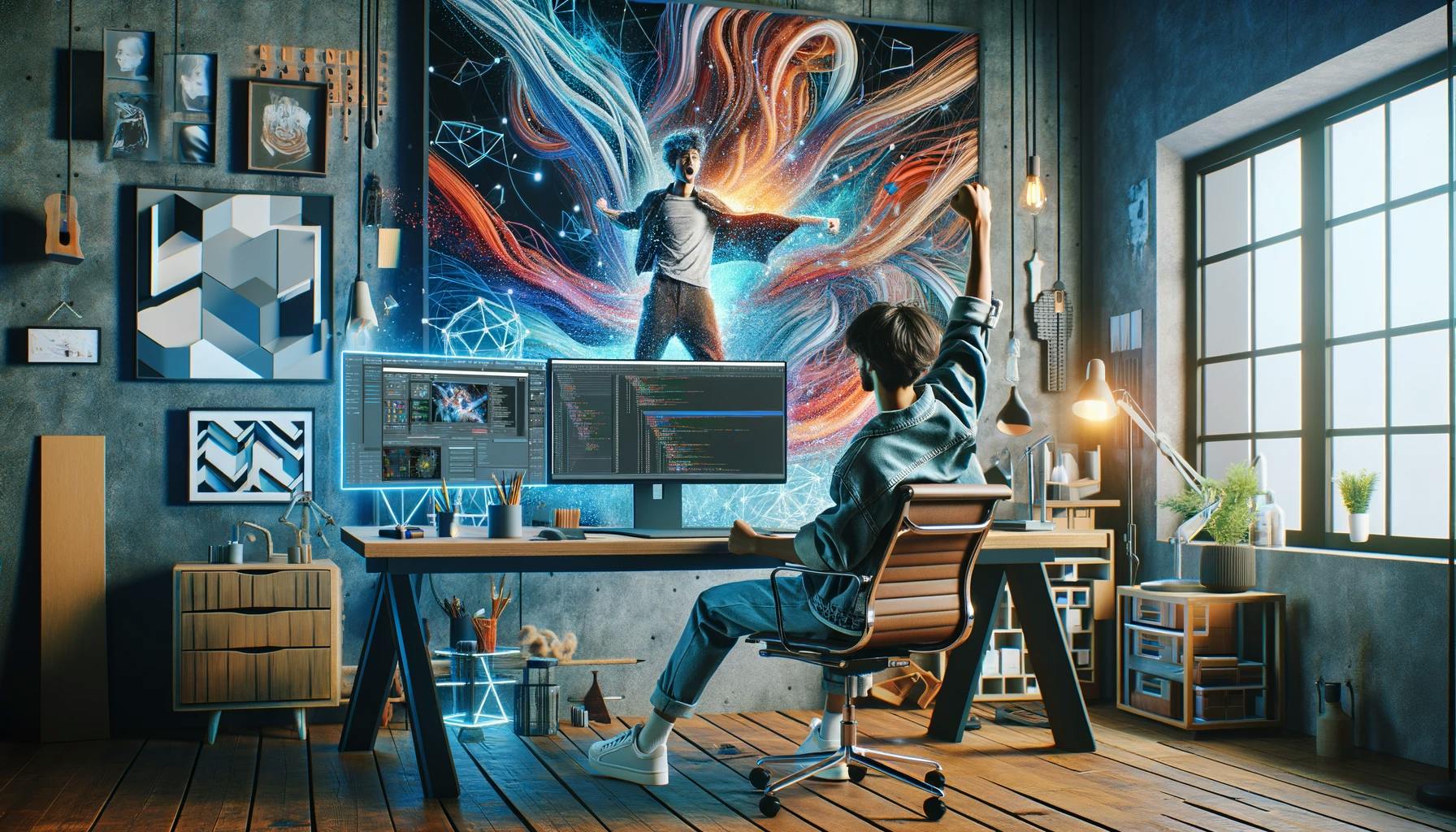
To add smooth transitions between your pages in a Svelte application, you can create a custom component that utilizes Svelte's transition
features. In this example, we'll use the slide
transition, but Svelte provides various transitions that you can explore.
PageAnimate
ComponentFirst, navigate to your src>lib
directory and create a new file named PageAnimate.svelte
. This component will be responsible for wrapping your page content and applying the transition effect.
Inside PageAnimate.svelte
, paste the following code:
<script>
import { slide } from 'svelte/transition';
</script>
<div transition:slide>
<slot />
</div>
This code imports the slide
transition from svelte/transition
and applies it to a <div>
element. The <slot />
tag is used to render any child content passed into the PageAnimate component.
Now, open the Svelte page file where you want to add the animation. In your case, it's the root +page.svelte
.
At the top of this file, import the PageAnimate
component using the following import statement:
import PageAnimate from "../lib/PageAnimate.svelte";
Then, wrap your main content or any other content you want to animate with the PageAnimate
component. For example:
<PageAnimate>
<main>
<!-- Your page content here -->
</main>
</PageAnimate>
By wrapping your content with PageAnimate
, the slide
transition will be applied whenever this component mounts or unmounts, giving your page a smooth sliding effect during navigation.