How to create a slider with splidejs
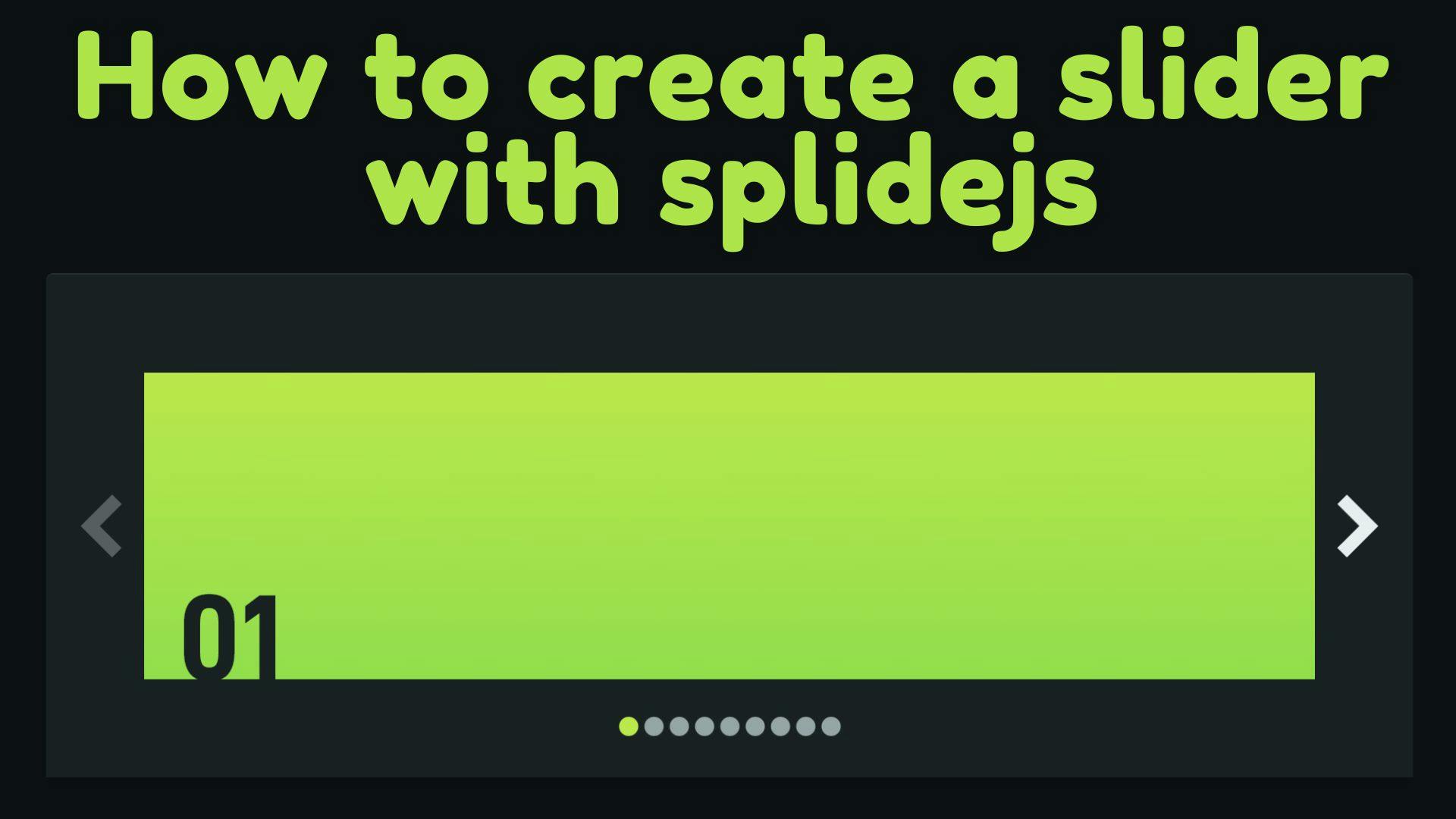
Slider is one of the most important components of a website, creating a slider is rather easy in sveltekit
npm i @splidejs/svelte-splide
slider
inside the lib
folder splidejs
import { Splide, SplideSlide } from "@splidejs/svelte-splide";
import "@splidejs/splide/dist/css/themes/splide-default.min.css";
export let Slide1 = "/sliderhome/desk_slider_A.jpg";
export let Slide1a = "/sliderhome/mob_slider_A.jpg";
export let Slide2 = "/sliderhome/desk_slider_B.jpg";
export let Slide2a = "/sliderhome/mob_slider_B.jpg";
With the script part of the slider complete, we must now focus on the markup section.
/sliderhome/desk_slider_A.jpg
means, I have placed the images insidestatic/sliderhome
folder
<Splide options={{"slider configuration"}} aria-label="sliderName">
<SplideSlide>
<div>
<!-- Placeholder of slider-->
</div>
</SplideSlide>
</Splide>
Based on this basic structure, here is my actual code<Splide
options={{
type: "fade",
heightRatio: 0.5,
pagination: false,
isNavigation: true,
arrows: true,
cover: true,
}}
aria-label="sliderName"
>
<SplideSlide>
<img src={Slide1} alt="image_alt" />
</SplideSlide>
<SplideSlide>
<img src={Slide2} alt="image_alt" />
</SplideSlide>
<SplideSlide>
<img src={Slide3} alt="image_alt" />
</SplideSlide>
<SplideSlide>
<img src={Slide4} alt="image_alt" />
</SplideSlide>
</Splide>
Our slider component is ready. To configure and customize the Splide slider, read all the options that Splide.js provides https://splidejs.com/guides/options/