Install Laravel with InertiaJS Svelte Tailwindcss DaisyUI
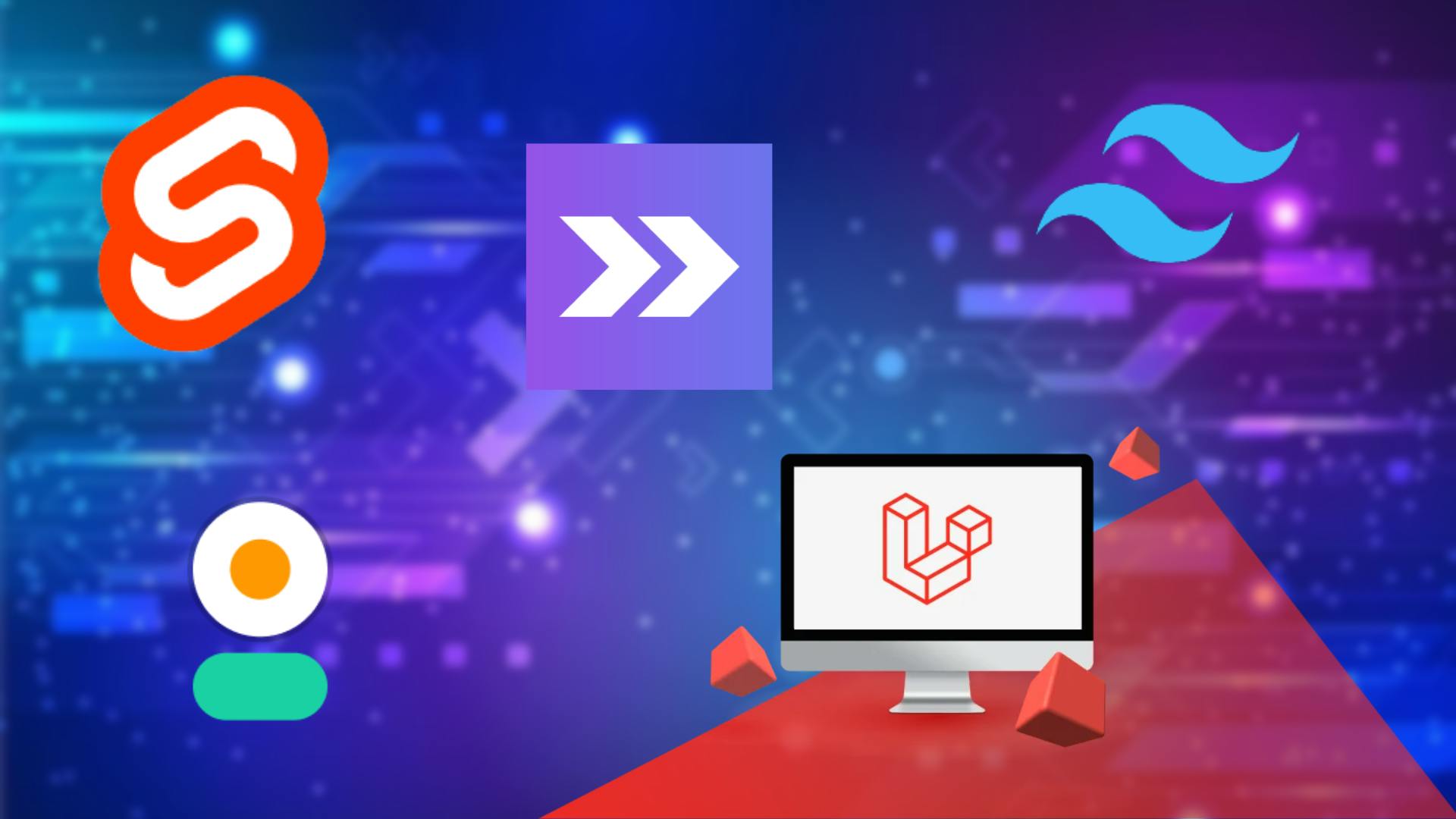
Create a folder and open CMD/Terminal with the folder location
composer create-project laravel/laravel .
This will create a Laravel Project inside the current directory
Now visit to InertiaJS website & let's do the server setup
composer require inertiajs/inertia-laravel
This will, install the Inertia server-side adapter using the Composer package manager.
Next, setup the root template that will be loaded on the first page visit to your application. So browse to laravel Resources>views
folder.
Create a file named app.blade.php
file & paste the below code snippet
<!DOCTYPE html>
<html>
<head>
<meta charset="utf-8" />
<meta name="viewport" content="width=device-width, initial-scale=1.0, maximum-scale=1.0" />
@vite('resources/js/app.js')
@inertiaHead
</head>
<body>
@inertia
</body>
</html>
Next we need to setup the Inertia middleware.
php artisan inertia:middleware
Once the middleware has been published,
register the HandleInertiaRequests
middleware in App\Http\Kernel.php
as the last item in your web
middleware group.
'web' => [
// existing code
\App\Http\Middleware\HandleInertiaRequests::class,
],
That's it, you're all ready to go server-side!
First, install the Inertia client-side adapter for svelte
npm install @inertiajs/svelte
Next, we have to update our main JavaScript file, which reside resources > js > app.js
to boot the Inertia app.
Copy the code snippet to resources > js > app.js
file
import "./bootstrap";
import { createInertiaApp } from "@inertiajs/svelte";
createInertiaApp({
resolve: (name) => {
const pages = import.meta.glob("./Pages/**/*.svelte", { eager: true });
return pages[`./Pages/${name}.svelte`];
},
setup({ el, App, props }) {
new App({ target: el, props });
},
});
Create a folder named Pages
inside resources/js
folder
Now create a file name Welcome.svelte
inside resources > js > Pages
folder
Now, copy the code snippet to Welcome.svelte
file
<script>
let stack = "svelte"
</script>
<main>
<h1>
Welcome to Laravel {stack} stack
</h1>
</main>
Open web.php
file inside routes folder
Now, we have to define the route to the file that we just created
<?php
use Inertia\Inertia;
use Illuminate\Support\Facades\Route;
Route::get('/', function () {
return Inertia::render('Welcome');
});
Now we have to install a package called @sveltejs/vite-plugin-svelte
which a vite plugin for svelte
npm install @sveltejs/vite-plugin-svelte
Now, open vite.config.js
file & insert this code inside plugin svelte({ })
so the final code will be
import { defineConfig } from "vite";
import laravel from "laravel-vite-plugin";
import { svelte } from "@sveltejs/vite-plugin-svelte";
export default defineConfig({
plugins: [
laravel({
input: ["resources/css/app.css", "resources/js/app.js"],
refresh: true,
}),
svelte({}),
],
});
Now we have to run two con-current command and both of this command must be run in separate window, you can not close the window at this moment
npm run dev
php artisan serve
Now we can see our svelte code running on http://127.0.0.1:8000/
and we can check vite configuration is working on http://localhost:5173/
At this point our setup is almost complete, and going forward is comparatively easy because a github user named tapan288 already published a composer package consist of laravel breeze with svelte component. Also this package take care of complex configuration of vite-svelte plugin and configuration of tailwind.
You can install the package via Composer:
composer require tapansharma/breeze-svelte --dev
Now install daisyui
npm i daisyui
open tailwind config file and add this line in plugin
require('daisyui')