Quickly add lightbox in sveltekit project using bigger-picture library
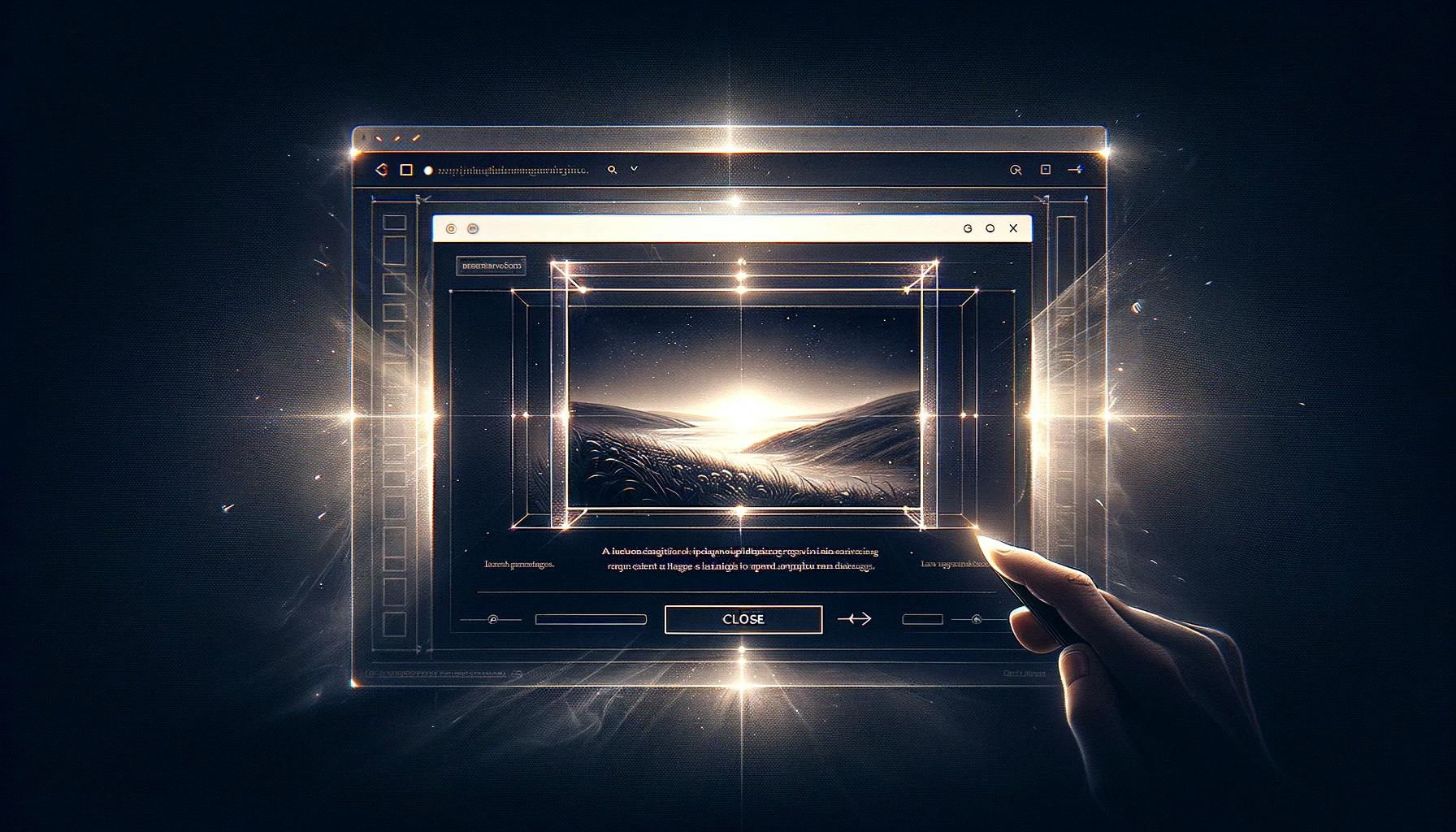
Let’s install the bigger-picture
npm package
npm install bigger-picture
bigger-picture
requires a specific type of HTML
Here is my markup code
<div
class="flex w-full flex-wrap justify-center gap-5 bg-[rgba(33,15,103,1)] p-5 pb-10"
>
{#each gallery as item}
<a class="block w-1/3 overflow-hidden shadow-xl md:w-1/4" href="/">
<img
class="h-full rounded-md object-cover"
src="{item.link}"
alt="{item.alt}"
/>
</a>
{/each}
</div>
The image must be inside an anchor tag; otherwise,
bigger-picture
won't be able to grab the click event to open the lightbox.
Now, let's import the bigger-picture
package in our lightbox component
import BiggerPicture from "bigger-picture/svelte";
import "bigger-picture/css";
Let’s initialize the bigger-picture
// For bigger picture
let bp;
let imageLinks;
function runBiggerPicture() {
let bp = BiggerPicture({
target: document.body,
});
}
onMount(() => {
runBiggerPicture();
});
bigger-picture
must be initialized after the DOM has loaded. In Svelte, this is achieved by invoking it within the onMount function
Let's adjust the markup to enable bigger-picture
to access specific image links.
<div
id="tris"
class="flex w-full flex-wrap justify-center gap-5 bg-[rgba(33,15,103,1)] p-5 pb-10"
>
{#each gallery as item}
<a
data-img="{item.link}"
data-thumb="{item.link}"
data-alt="image description"
class="block w-1/4 overflow-hidden shadow-xl"
href="/"
>
<img
class="h-full rounded-md object-cover"
src="{item.link}"
alt="{item.alt}"
/>
</a>
{/each}
</div>
Next, we'll select all anchor elements containing images within the tris
div.
// initialize
function runBiggerPicture() {
bp = BiggerPicture({
target: document.body,
});
// Bigger Picture - grab image links
imageLinks = document.querySelectorAll("#tris > a");
imageLinks.forEach((imageLink) => {
imageLink.addEventListener("click", openGallery);
});
}
In the following code, we are calling the openGallery function when the user clicks on the imageLink, so let’s write the openGallery function.
// BiggerPicture - function to open function
openGallery(e) {
e.preventDefault();
bp.open({
items: imageLinks,
el: e.currentTarget
});
}
Let's take a look at the complete code
<script>
import { onMount } from "svelte";
import { OurDhakaGallery } from "./../common_data/ImageGallery.js";
let title = `Our Dhaka`;
let { gallery } = OurDhakaGallery;
console.log(gallery);
onMount(() => {
runBiggerPicture();
});
// bigger picture
import BiggerPicture from "bigger-picture/svelte";
import "bigger-picture/css";
// For bigger picture
let bp;
let imageLinks;
// initialize bigget picture
function runBiggerPicture() {
bp = BiggerPicture({
target: document.body,
});
// Bigger Picture - grab image links
imageLinks = document.querySelectorAll("#tris > a");
imageLinks.forEach((imageLink) => {
imageLink.addEventListener("click", openGallery);
});
}
// BiggerPicture - function to open
function openGallery(e) {
e.preventDefault();
bp.open({
items: imageLinks,
el: e.currentTarget,
});
}
</script>
<div class=" bg-[rgba(33,15,103,1)] px-32 pb-5 pt-10 text-center text-xl">
<div
class="prose mx-auto max-w-none px-32 text-center font-Siliguri text-xl text-white prose-headings:text-white"
>
<h1>{title}</h1>
</div>
</div>
<div
id="tris"
class="flex w-full flex-wrap justify-center gap-5 bg-[rgba(33,15,103,1)] p-5 pb-10"
>
{#each gallery as item}
<a
data-img="{item.link}"
data-thumb="{item.link}"
data-alt="image description"
class="block w-1/3 overflow-hidden shadow-xl md:w-1/4"
href="/"
>
<img
class="h-full rounded-md object-cover"
src="{item.link}"
alt="{item.alt}"
/>
</a>
{/each}
</div>