Seamlessly Integrating MongoDB with SvelteKit Using Prisma: A Comprehensive Guide
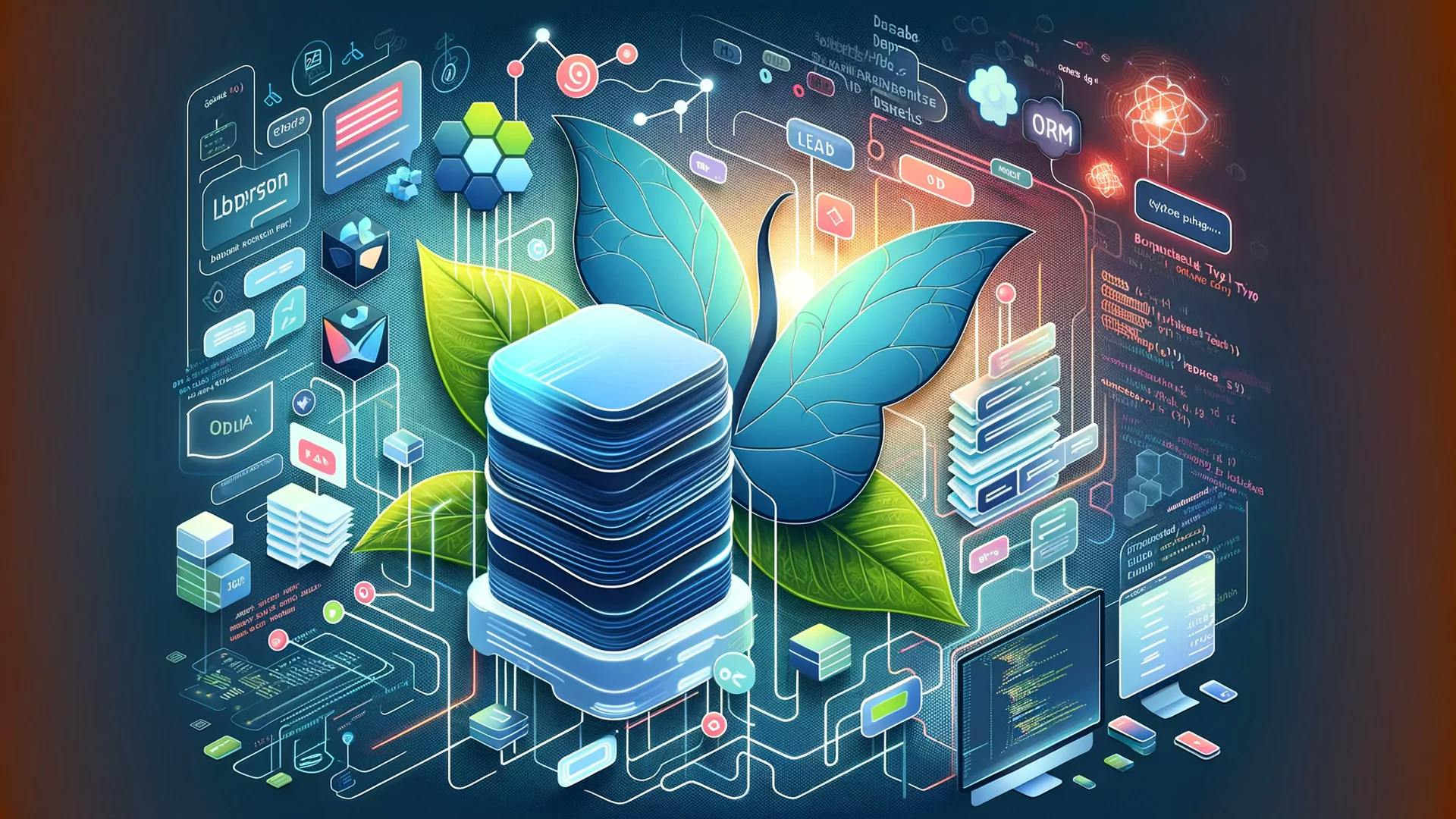
.env
file in the root directory and paste the following code:DATABASE_URL =
"mongodb+srv://momo:<password>@momo.xogao6r9.mongodb.net/momo?retryWrites=true&w=majority";
npm install mongodb
npm i prisma -D
npm i @prisma/clients
npx prisma init
This command creates a prisma
folder in the root directory along with a schema.prisma
file. schema.prisma
and add the following configuration:generator client {
provider = "prisma-client-js"
}
datasource db {
provider = "mongodb"
url = env("DATABASE_URL")
}
for schema formatting Prisma - Visual Studio Marketplace
to directly interact with your database. MongoDB for VS Code - Visual Studio Marketplace
mongodb+srv://momo:<password>@momo.xogao6r9.mongodb.net/
momo
. schema.prisma
to define your data model:model opinion {
id String @id @default(auto()) @map("_id") @db.ObjectId
type String
vote Int
}
Then, generate the Prisma client:npx prisma generate
+page.server.js
, add the following code to interact with the database:import { PrismaClient } from "@prisma/client";
const prisma = new PrismaClient();
async function mainA() {
const creatingData = await prisma.opinion.create({
data: {
vote: 1117,
type: "kabooz",
},
});
console.log(creatingData);
}
mainA();