SvelteKit Forms Simplified: Mastering Minimal Setup for Robust Actions
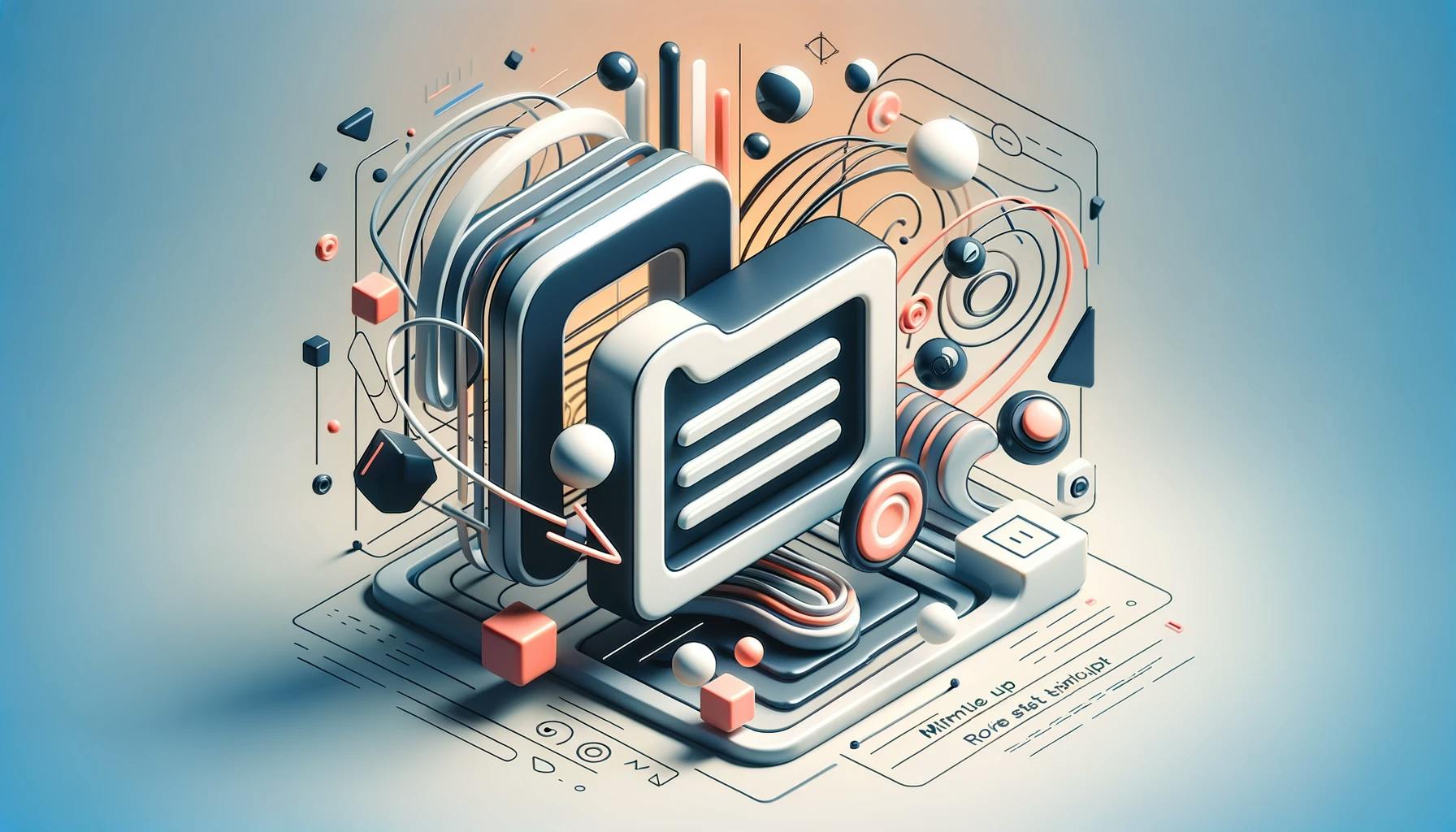
In web development, forms
are essential for user interactions, from sign-ups to feedback submissions. As developers aim for more efficient web applications, the need for streamlined form handling is crucial. SvelteKit, a modern framework, offers a simplified yet powerful approach to form management through unnamed
and named actions
.
This guide explores SvelteKit's form handling, providing insights for both beginners and seasoned developers to enhance server interactions and user experiences.
Svelte-Kit offers two types of form handling mechanisms:
named
andunnamed/ default
actions.
Unnamed actions are a default
handling method where the form does not specify a particular action, submitting data to the same endpoint instead.
Named actions, on the other hand, allow you to direct the form submission to specific endpoints by adding a query parameter with a name. This method provides more control and is ideal for handling different forms or submission types on the same page, making it a versatile choice for more complex scenarios.
Let's take a look at unnamed actions in detail
form
tag name
property form
must have method="POST"
submit
button. type=submit
not required <main class="pb-20">
<div
class="navbar border-b-2 border-gray-500 border-opacity-25 bg-base-100"
>
<h1 class="text-xl font-bold uppercase">Provide your name and email</h1>
</div>
<form method="post">
<div class="flex flex-col gap-4 p-4">
<!-- name input field -->
<input
name="name"
type="date"
placeholder="Date"
class="input input-bordered input-primary w-full outline-none focus:border-none"
/>
<!-- email input field -->
<input
name="email"
type="number"
placeholder="Total Active Seconds"
class="input input-bordered input-primary w-full outline-none focus:border-none"
/>
<div class="flex w-full flex-row gap-5">
<button class="btn btn-primary flex-grow">Submit</button>
</div>
</div>
</form>
</main>
I have used Tailwind CSS and DaisyUI, which are utility-first CSS framework and component library respectively, to style the following code.
export const actions = {
default: async ({ request }) => {
const data = await request.formData();
const email = data.get("email");
const name = data.get("name");
return { success: true };
},
};
+page.svelte
file we need to declare export let form
in the +page.svelte
file to access the form submission results export const actions = {
default: async ({ request }) => {
const formData = Object.fromEntries(await request.formData());
console.log(formData);
return {
success: true,
formData: formData,
};
},
};
<script>
export let form;
console.log(form);
</script>
input field
must be wrapped with a form
tag input field
must have name
property method=”POST”
& action property action="?/register"
?/
characteraction = "?/register";