Sveltekit Pagination with Prismic
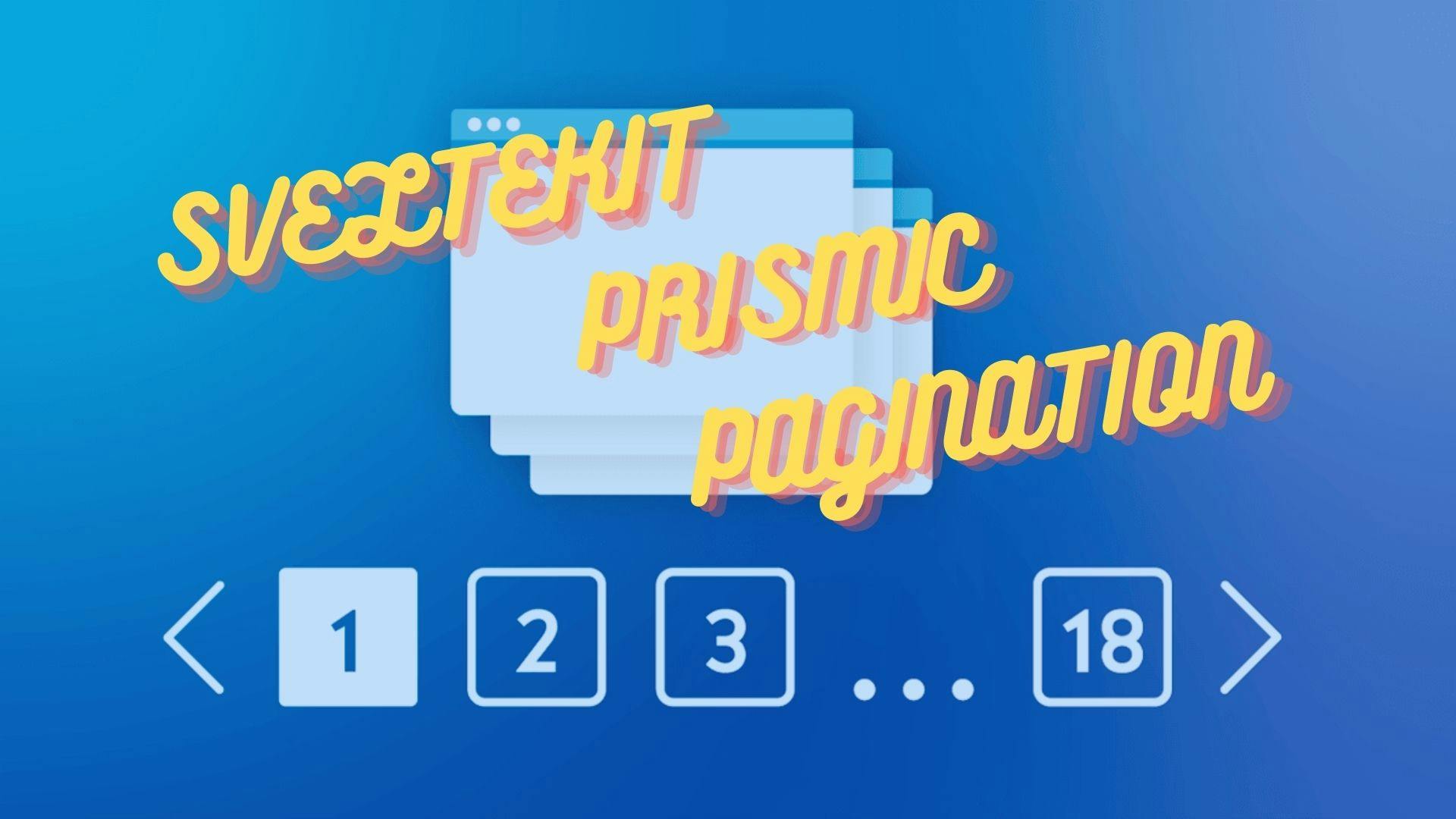
By default, SvelteKit will return 50 post responses if no specific instructions are provided regarding the number of results to be returned.
Below is the code snippet that I have written for my website...
import createClient from '$lib/prismicio';
export async function load({ fetch, request, params }) {
const client = createClient({ fetch, request });
const data = await client.getByType('blog', {
orderings: {
field: 'document.first_publication_date',
direction: 'desc'
},
pageSize: 4,
page: 1
});
if (data) {
return {
data: data,
};
}
}
It appears that in my case, I have set a fixed page number which is (page:1) and specified that, I want to limit the number of responses (pageSize: 4) to four per API call.
So our first step will be to build a url with the search query parameter. Sveltekit allows us to do that easily by adding a ‘?’ question mark, followed by a search query text, and then assigning any variable to it.
So the final structure will be something like this
www.sabbirz.com/blog?page=1
Now we can update our fetch query
import createClient from '$lib/prismicio';
export async function load({ fetch, request, url }) {
const client = createClient({ fetch, request });
let pageNum = url.searchParams.get('page');
if (!pageNum) {
pageNum = 1;
}
const data = await client.getByType('blog', {
orderings: {
field: 'document.first_publication_date',
direction: 'desc'
},
pageSize: 4,
page: pageNum
});
if (data) {
return {
data
};
}
}
This should solve the pagination problem
But we haven’t yet written any pagination frontend html code.
Let’s do that by inspecting the js object from prismic
Here we can see we have some important information here, like
Next_page url, prev_page url, total post as total_results_size, results_per_page, etc.
Based on that info we can build a pagination component, here is mine
<script>
export let length = 2;
export let currentPage = 3;
</script>
<div class=" text-center pt-5">
<div class="btn-group">
<a class="btn btn-outline" href="?page={currentPage - 1 <= 0 ? length : currentPage - 1}"
>Prev</a
>
{#each { length: length } as _, i}
<!-- {i} -->
<a
href="?page={i + 1}"
class="btn {i + 1 == currentPage ? 'btn-secondary' : 'btn-outline'} ml-[.5px]">{i + 1}</a
>
{/each}
<a class="btn btn-outline" href="?page={currentPage + 1 > length ? '1' : currentPage + 1}"
>Next</a
>
</div>
</div>