Solving the Unintended Form Submission Dilemma Using A Simple HTML Button Type Fix
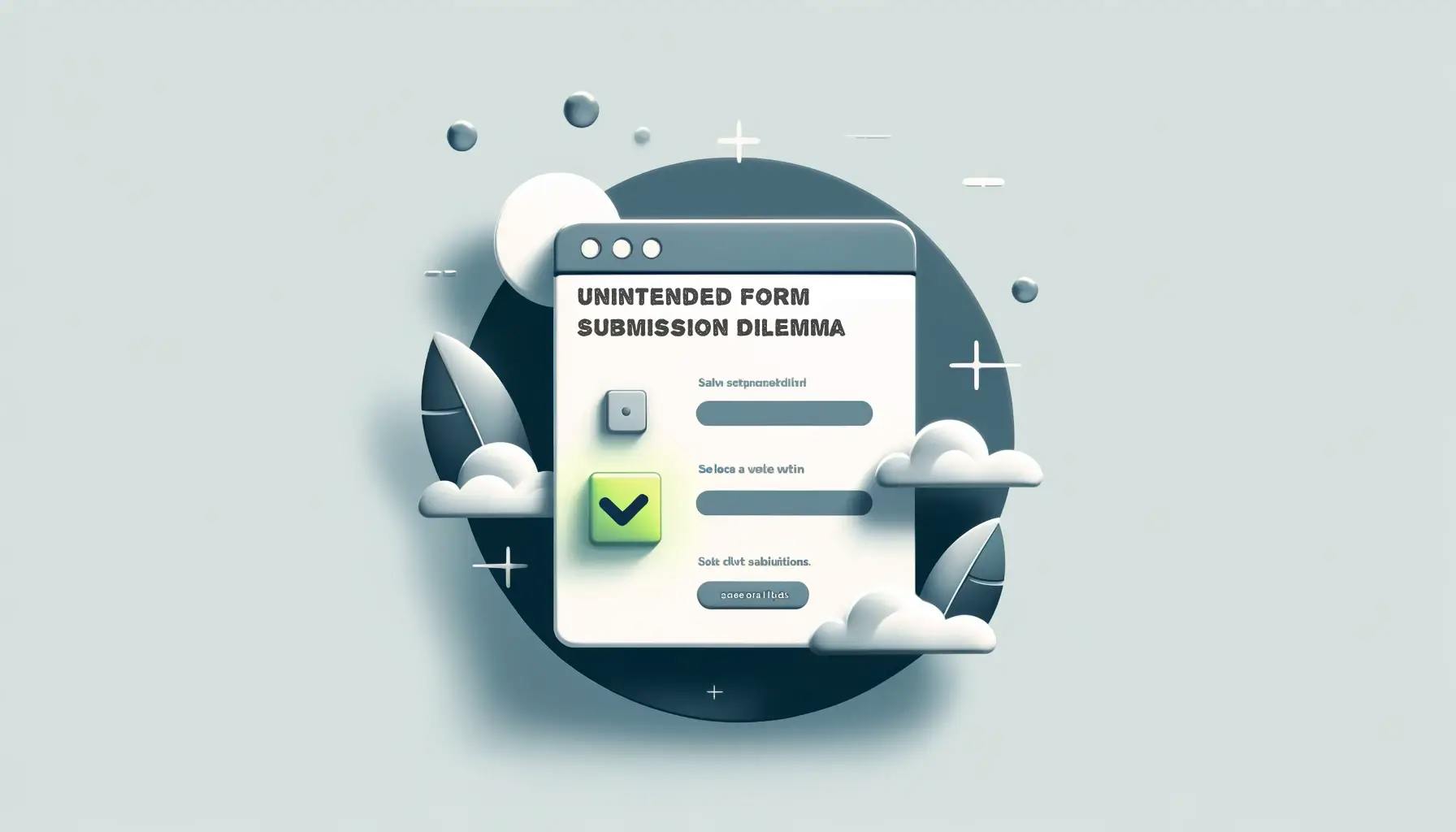
When developing form
s, it's crucial to understand how different elements interact within the form
, particularly button
s.
A common oversight is the unintentional form
submission triggered by button
s, which can lead to data loss or unexpected behavior.
This post delves into this issue and provides a straightforward solution that is applicable across various web development frameworks, including SvelteKit.
When you place a button inside a form, its default behavior is to submit the form unless you explicitly handle the button's action. This can lead to unintended form submissions, if not managed correctly.
Consider the following code snippet, which illustrates this issue:
<script>
function formResetter() {
console.log('function called');
Object.keys($form).forEach((key) => {
$form[key] = ''; // Set each key to an empty string
});
}
</script>
<form method="post">
<div class="flex w-full flex-row gap-5">
<button class="btn btn-primary flex-grow">Submit</button>
<!-- This button unintentionally submits the form -->
<button
on:click={formResetter}
class="btn btn-square btn-outline btn-primary"
>
Run Function Button
</button>
</div>
</form>
In this code snippet, both
button
s are within a<form>
element. The secondbutton
, intended to run a JavaScript function without submitting theform
, but thisbutton
triggers aform
submission. This happens because, by default,button
s within aform
are of typesubmit
, causing theform
tosubmit
when clicked.
The solution to this problem is surprisingly simple—explicitly define the button
's type
attribute as button
:
<script>
function formResetter() {
console.log('function called');
Object.keys($form).forEach((key) => {
$form[key] = ''; // Set each key to an empty string
});
}
</script>
<form method="post">
<div class="flex w-full flex-row gap-5">
<button class="btn btn-primary flex-grow">Submit</button>
<button
type="button"
on:click={formResetter}
class="btn btn-square btn-outline btn-primary"
>
Run Function Button
</button>
</div>
</form>
By setting type="button"
, we instruct the browser that this button
's purpose is not to submit
the form
, thus preventing unintended form
submissions when it's clicked. This minor yet impactful change ensures that the button fulfills its intended function without interfering with the form
's behavior.
While the solution is straightforward, understanding the underlying mechanics is beneficial for web developers. In HTML form
s, the default type for a button
is submit
, which means without specifying the type, any button
within a form will submit
it upon being clicked. By explicitly setting the type to button
, we override this default behavior, making our intentions clear both to the browser and to anyone else reading the code.
This practice is not just limited to SvelteKit but is applicable in any HTML-based web development framework. It highlights the importance of being explicit about element behaviors in your code, ensuring that each element behaves as intended.